TauriはバックエンドにRust、フロントエンドには従来のWeb開発技術(JavaScript+HTML+CSS,ReactやNext.jsなどのフレームワーク)を用いたデスクトップアプリケーションを開発するためのフレームワークです。
クロスプラットフォーム対応しておりWindowsでもMacでもLinuxでも動作します。
iOS/Androidへの対応も現在進行系で進んでおりAlpha Releaseとして公開されています。
そんなTauriですが2022年6月に待望のバージョン1.0がリリースされました。
ということで今回はTauri+Next.jsな環境を構築してみたいと思います。
- Tauri+Next.jsの開発環境構築
- フロントエンド(TypeScript)からバックエンド(Rust)の処理を呼び出す
前提条件
本記事で紹介する環境は、Node.js(npm)およびRustが実行できることを前提としています。
Rustのインストールに関してはこちらの記事でも紹介していますのでご覧ください。
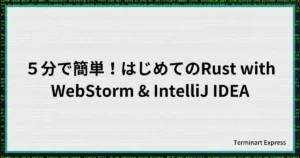
プロジェクトの作成
まずはじめにプロジェクト全体のセットアップをします。
mkdir projectname
作成したディレクトリ内で下記コマンドを実行して初期化をしておきましょう。
npm init
設定は基本的にすべてデフォルトでOKです。
-zsh % npm init
...中略
package name: (sample)
version: (1.0.0)
description:
entry point: (index.js)
test command:
git repository:
keywords:
author:
license: (ISC)
以降は上記にて作成したフォルダをルートフォルダとします。
フロントエンド(Next.js)の作成
次にフロントエンド部分(Next.js)を作成していきます。
下記のコマンドを実行してコード生成を行います。
npx create-next-app@latest --use-npm --typescript
入力項目は以下のようにします。
-zsh % npx create-next-app@latest --use-npm --typescript
✔ What is your project named? … next
✔ Would you like to use ESLint with this project? … Yes
✔ Would you like to use `src/` directory with this project? … Yes
✔ Would you like to use experimental `app/` directory with this project? … No
Creating a new Next.js app in /sample/next.
...中略
Success! Created next at /sample/next
Tauri向けにnext.config.js
の設定を下記のように修正します。
/** @type {import('next').NextConfig} */
const nextConfig = {
reactStrictMode: true,
swcMinify: true,
// Note: This experimental feature is required to use NextJS Image in SSG mode.
// See https://nextjs.org/docs/messages/export-image-api for different workarounds.
images: {
unoptimized: true,
},
}
module.exports = nextConfig
スクリプトをnext/package.json
に追加します。
{
...
"scripts": {
"dev": "next dev",
"build": "next build",
"export": "next export",
"start": "next start",
"lint": "next lint"
},
...
}
バックエンド(Tauri)の作成
続いて今回のメインであるバックエンド部分(Tauri)を作成します。
ルートフォルダで以下コマンドを実行します。
npm install --save-dev @tauri-apps/cli
スクリプトを/package.json
に追加します。
{
...
"scripts": {
...
"tauri": "tauri",
...
},
...
}
下記コマンドを実行してTauriの初期セットアップを行います。
npm run tauri init
コマンド実行後の質問に対しては以下のように答えていきます。
✔ What is your app name? · projectname (任意のアプリ名を入力)
✔ What should the window title be? · titile (任意のタイトルを入力)
✔ Where are your web assets (HTML/CSS/JS) located, relative to the "<current dir>/src-tauri/tauri.conf.json" file that will be created? · ../out
✔ What is the url of your dev server? · http://localhost:3000
✔ What is your frontend dev command? · npm run dev
✔ What is your frontend build command? · npm run build && npm run export
ビルド関連の設定を修正します。
tauri.conf.json
のbuild
部分を以下のように修正します。
{
...
"build": {
"beforeBuildCommand": "npm run build && npm run export",
"beforeDevCommand": "npm run dev",
"devPath": "http://localhost:3000",
"distDir": "../out"
},
...
}
スクリプトの最終調整
以下のように/package.json
を修正します。
{
...
"scripts": {
"build": "npm run build --prefix next",
"dev": "npm run dev --prefix next",
"export": "npm run export --prefix next",
"tauri": "tauri"
},
...
}
以下のようにnext/package.json
を修正します。
{
...
"scripts": {
"dev": "next dev",
"build": "next build",
"export": "next export -o ../out",
"start": "next start",
"lint": "next lint"
},
...
}
動作確認
下記コマンドをルートで実行して動作すればひとまず問題なしです!!
npm run tauri dev
Rust部分でエラーが発生した場合、
- Rustをアップデートする
- stableバージョンを使う
などを行うと解消できる可能性が高いです。
参考)関連コマンド
rustup self update ・・・ rustupのアップデート
rustup default stable ・・・ 使用するRustのデフォルトバージョンをstableにする
rustup update ・・・ 使用中のRustバージョンをアップデートする
おまけ:Tauriフォルダ名の変更
Tauri部分のフォルダ名はデフォルトではsrc-tauri
となっています。
個人的に気になったのでtauri
というフォルダ名に変更。
動作確認したところ正常に動作することも確認できました。
IDEのリファクタリング機能で簡単にできますので気になる方は変更しておきましょう。
フロントエンド(TypeScript)からバックエンド(Rust)のAPIコール
以下を/next
配下へインストール
npm i @tauri-apps/api
tauri/src/main.rs
を以下のように編集
#![cfg_attr(
all(not(debug_assertions), target_os = "windows"),
windows_subsystem = "windows"
)]
fn main() {
tauri::Builder::default()
.invoke_handler(tauri::generate_handler![greet])
.run(tauri::generate_context!())
.expect("error while running tauri application");
}
#[tauri::command]
fn greet(name: &str) -> String {
format!("Hello, {}!", name)
}
next
をビルドしていない場合に以下のエラーが出る場合があります。
The `distDir` configuration is set to `"../out"` but this path doesn't exist
その場合はルートフォルダで下記のコマンドを実行しましょう。
npm run export
next/src/pages/index.tsx
を以下のように編集します。
import {invoke, InvokeArgs} from '@tauri-apps/api/tauri';
import {useEffect} from 'react';
function callTauriApi(name: string, args: InvokeArgs) {
const isClient = typeof window !== 'undefined'
if (isClient) {
invoke(name, args).then(console.log).catch(console.error)
}
}
const Home = () => {
useEffect(() => {
callTauriApi('greet', { name: 'World' })
}, [])
return (
<div>
<label>api test</label>
</div>
)
}
export default Home
ルートフォルダで以下コマンドを実行します。
npm run tauri dev
エラーなく動作すればデスクトップアプリケーションが起動します。
コンソール画面を開いて以下のように表示されれば問題なしです!
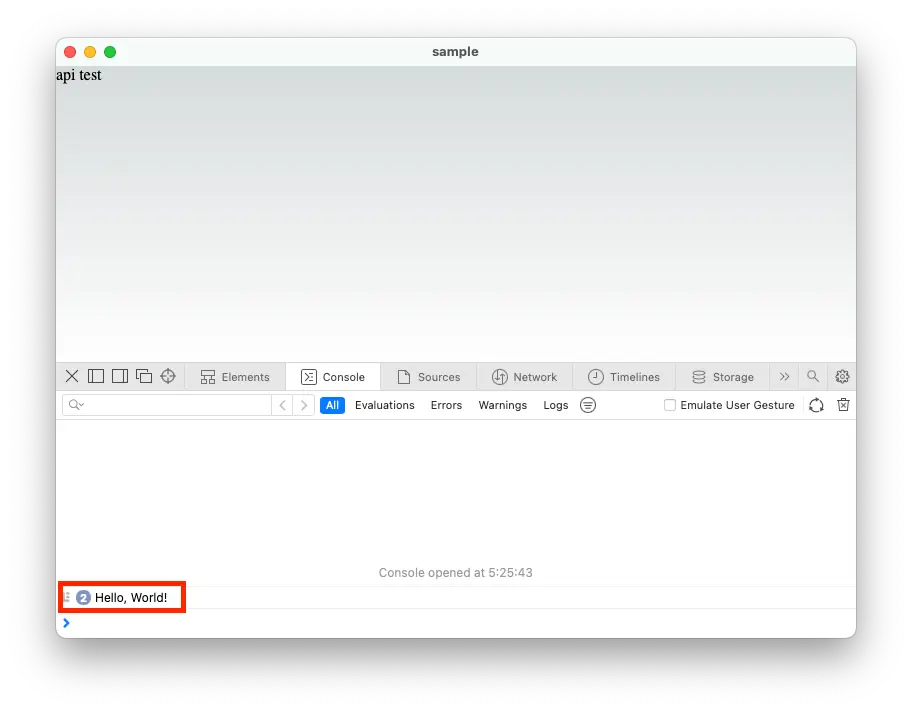
最後に
従来のフロントエンド技術に加えて、強力な型付け言語であるRustを使用できるTauri。
Rustは他言語と異なる部分も多く難しい言語だとは思いますが、その分より安全な実装をすることができますのでバックエンドにはもってこいです。
TauriもRustもどんどんアップデートが進んでおり将来への期待が大きいのも良いところ。
今回紹介したように環境構築が簡単ですので試しにTauriに触れてみてはいかがでしょうか。